Custom map simulations¶
In this tutorial we will build a simulation from scratch.
We start by defining a Band
that will determine our array’s sensitivity to different spectra. We then generate an array by specifying a field of view, which will be populated by evenly-spaced beams of the given band.
[1]:
import maria
from maria.instrument import Band
f090 = Band(
center=90, # in GHz
width=20,
sensitivity=3e-5, # in K sqrt(s)
knee=1e0,
gain_error=5e-2,
)
f150 = Band(center=150, width=30, sensitivity=5e-5, knee=1e0, gain_error=5e-2)
[2]:
array = {"field_of_view": 0.5, "primary_size": 20, "bands": [f090, f150]}
instrument = maria.get_instrument(array=array)
instrument.plot()
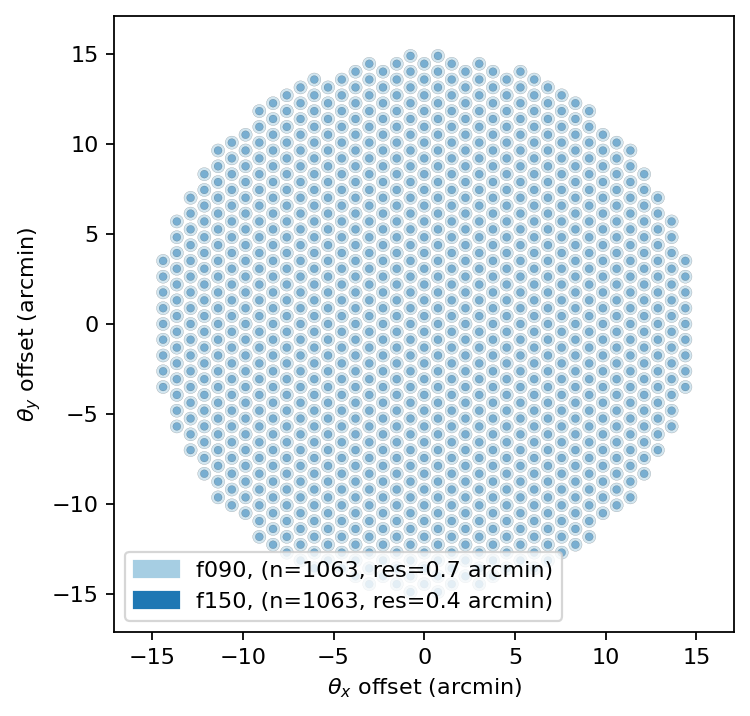
As something to observe, we can download a map and construct a map
. We also define a plan to do a daisy scan centered on the center of the map.
[3]:
from maria.io import fetch
map_filename = fetch("maps/big_cluster.fits")
input_map = maria.map.read_fits(
filename=map_filename, nu=150, index=1, width=1, center=(150, 10), units="Jy/pixel"
)
input_map.data *= 1e1
input_map.to(units="K_RJ").plot()
2024-12-14 03:51:48.501 INFO: Caching data from https://github.com/thomaswmorris/maria-data/raw/master/maps/big_cluster.fits
100%|██████████| 4.20M/4.20M [00:00<00:00, 94.1MB/s]
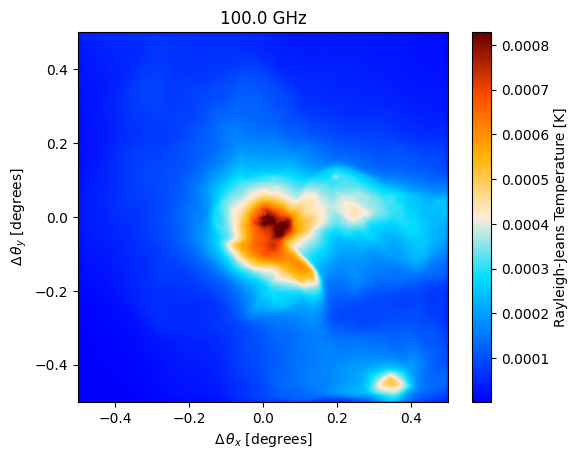
[4]:
plan = maria.Plan(
scan_pattern="daisy",
scan_options={"radius": 0.4, "speed": 0.1}, # in degrees
duration=600, # in seconds
sample_rate=50, # in Hz
scan_center=(150, 10),
frame="ra_dec",
)
plan.plot()
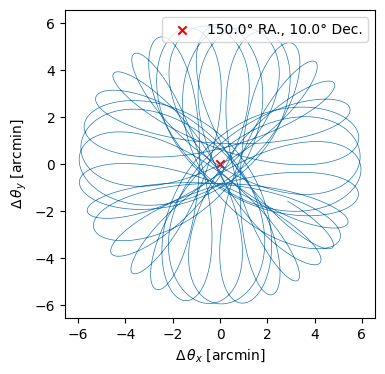
[5]:
sim = maria.Simulation(
instrument,
plan=plan,
site="llano_de_chajnantor",
map=input_map,
atmosphere="2d",
)
2024-12-14 03:51:57.274 INFO: Initialized base in 7513 ms.
Building atmosphere: 100%|██████████| 6/6 [00:33<00:00, 5.61s/it]
2024-12-14 03:52:33.887 INFO: Initialized atmosphere in 36581 ms.
[6]:
tod = sim.run()
Generating turbulence: 100%|██████████| 6/6 [00:00<00:00, 81.41it/s]
Sampling turbulence: 100%|██████████| 6/6 [00:07<00:00, 1.32s/it]
Computing atmospheric emission: 100%|██████████| 2/2 [00:02<00:00, 1.14s/it, band=f150]
Computing atmospheric opacity: 100%|██████████| 2/2 [00:02<00:00, 1.17s/it, band=f150]
Sampling map: 100%|██████████| 2/2 [00:28<00:00, 14.08s/it, band=f150]
Generating noise: 100%|██████████| 2/2 [00:03<00:00, 1.77s/it, band=f150]
[7]:
from maria.mappers import BinMapper
mapper = BinMapper(
center=(150, 10),
frame="ra_dec",
width=1e0,
height=1e0,
resolution=5e-3,
tod_preprocessing={
"window": {"name": "tukey", "kwargs": {"alpha": 0.1}},
"despline": {"knot_spacing": 5},
"remove_modes": {"modes_to_remove": [0]},
},
map_postprocessing={
"gaussian_filter": {"sigma": 1},
"median_filter": {"size": 1},
},
)
mapper.add_tods(tod)
output_map = mapper.run()
Running mapper (f090): 100%|██████████| 1/1 [00:23<00:00, 23.27s/it]
Running mapper (f150): 100%|██████████| 1/1 [00:22<00:00, 22.76s/it]
[8]:
output_map.plot()
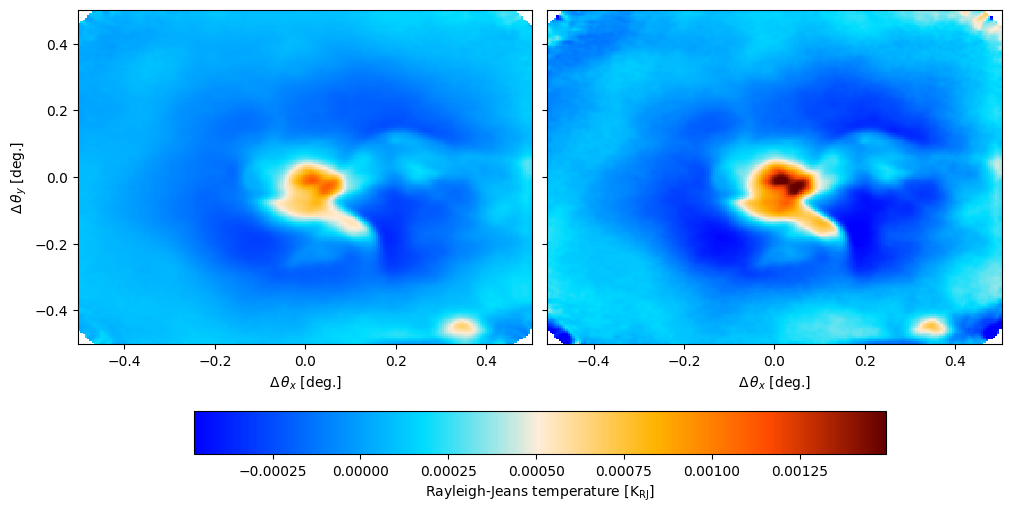